Email HTMLbody and HTML Filler Object
Email HTMLbody, HTML
The HTMLFiller object is used to fill bookmarked locations in a HTML document – the HTMLBody property of an email or an HTML page containing with data. The HTMLFiller is added to your VBA project when you create an Outlook email process using Document Creation using Microsoft Access.
Bookmarks
Bookmarks normally correspond to the name of the field or control that provides the data.
Bookmarks are used in two ways:
- strings like [CompanyID] with square brackets surrounding the bookmarkname are simply intended to be replaced by a data value.
- The value of id attributes for identifying more complex elements such as an image, a list or a table.
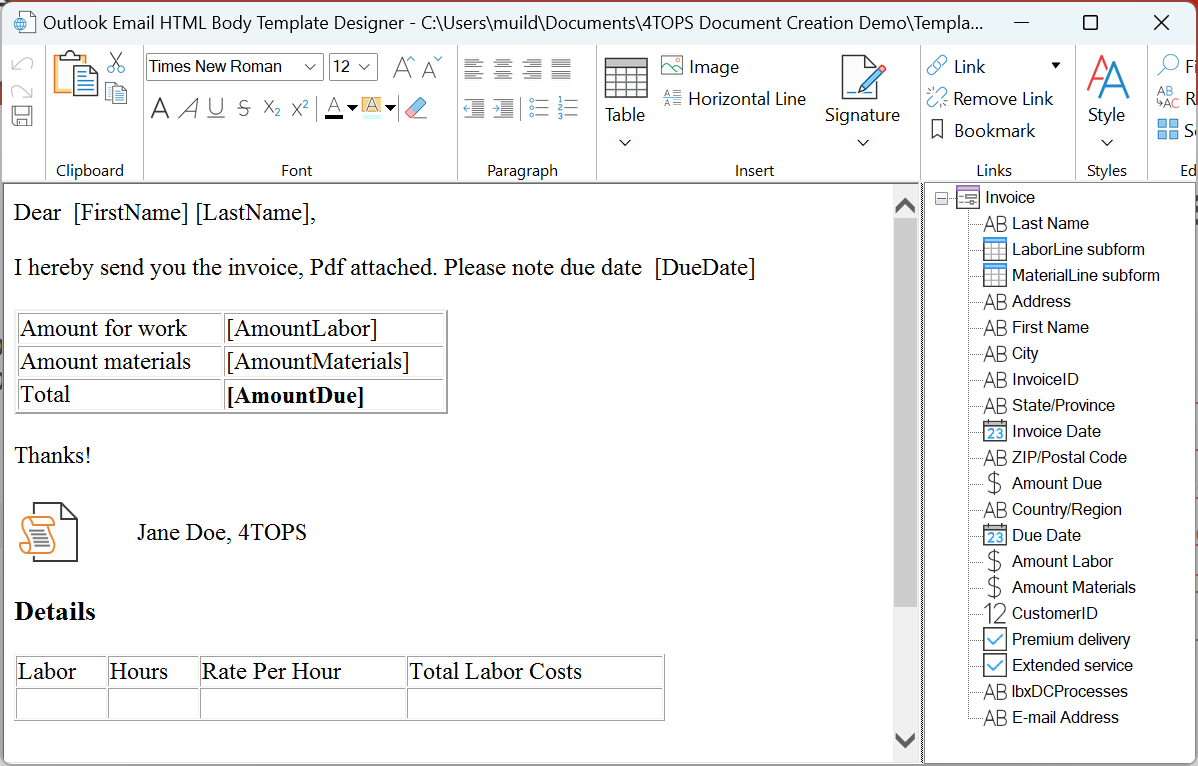
Set HTMLBody from template filled with data
Dim appOutlook As Outlook.Application
Set appOutlook = New Outlook.Application
Dim mimEmail As Outlook.MailItem
Set mimEmail = appOutlook.CreateItem(olMailItem)
Dim hfr As New HTMLFiller
hfr.Load FileName:=strTemplateFile
hfr.FillPicture Bookmark:="Image", ImageFile:=ControlValue(control:=![Image])
hfr.InsertFile Bookmark:="HTMLFile", FileName:=ControlValue(control:=![HTMLFile])
hfr.FillElement Bookmark:="Usage", Value:=ControlValue(control:=![Usage])
hfr.FillListFromArray Bookmark:="ListofReasonssubform", Array1D:=ValuesInSubFormColumn( _
control:=![List of Reasons subform],ColumnControlName:="Reason")
mimEmail.HTMLBody = hfr.HTML
Methods
Most methods below concern different ways data is used to fill areas in the template HTML file on bookmarked locations. For example in case of an Image element <img> with id ‘myimage’ the supplied data will be used to fill the source src attribute with the file location of the picture.
AddHyperlink
Inserts a hyperlink at the bookmarked location.
Public Sub AddHyperlink(Bookmark As String, Value As Variant, Optional ParseValue As Boolean = False, _
Optional TextToDisplay As String, Optional SubAddress As String)
- Bookmark: uses text string [mybookmark]
- Value: Both text and hyperlink fields can be used as the source. In the former case the link address and the displayed text are equal, e.g.
<a href="https://www.4tops.com">https://www.4tops.com/</a>
. With hyperlink fields the input has a syntax using # as separator: TextToDisplay#Address#SubAddress#ScreenTip where only the Address is mandatory. AddHyperlink is capable of translating this to a HTML Hyperlink <a> element. - ParseValue: The # convention in hyperlink fields may also be used when supplying text values, e.g.
That's us #https://www.4tops.com/
– for this you need in the email creation process VBA code specifyParseValue:=True
as extra argument. - TextToDisplay: Optionally set the display text for the hyperlink.
- SubAddress: In case you want to point to a bookmark on the page, e.g.
https://www.4tops.com/
#mybookmark.
ApplyBoolean
If True the value CHECKED is added to the INPUT type=checkbox
element – showing a checked checkbox. If False CHECKED is removed, making it unchecked, see forms – What’s the proper value for a checked attribute of an HTML checkbox? – Stack Overflow.
Public Sub ApplyBoolean(Bookmark As String, Value As Variant)
- Bookmark: id attribute in
<INPUT type=checkbox>
element - Value: an expression to supply a Boolean value
True
orFalse
FillElement
Replaces all instances with the bookmark with the string value. This concerns both the visible text and html element attributes.
Public Sub FillElement(Bookmark As String, Value As String)
- Bookmark: [bookmarkname] – between square brackets
- Value: any value presentable as text
FillListFromArray
Adds the items in the 1-dimensional array in list item elements <li>
Public Sub FillListFromArray(Bookmark As String, Array1D As Variant)
- Bookmark: [bookmarkname] – between square brackets
- Array1D: A 1-dimensional array
FillPicture
Sets the source attribute of the <IMG>
element with the filepath specified in ImageFile argument. This will replace the dummy image previously set in the template.
Public Sub FillPicture(Bookmark As String, ImageFile As String)
- Bookmark: id attribute in <IMG id=MyImage src= > element
- ImageFile: the picture’s path and file name
FillPictureFromAttachment
Same as FillPicture, but now obtaining the picture from the Attachment.
Public Sub FillPictureFromAttachment(Bookmark As String, control As Attachment)
- Bookmark: id attribute in <IMG id=MyImage src= > element
- Control: a field or control of type Attachment containing the picture
FillTableFromArray
Fill Table From Array. Bookmark = Table.Id
Public Sub FillTableFromArray(Bookmark As String, Array2D As Variant, Optional ColumnOrder As String)
- Bookmark: id attribute in
<Table>
element - Array2D: 2-dimensional array
- ColumnOrder: the order in which the columns in the input array are placed in the HTML table, for additional flexibility.
InsertFile
Inserts the contents of another HTML file at the bookmark indicated an <IMG>
element. The dummy text file image indicating the location is removed in the process.
Public Sub InsertFile(Bookmark As String, FileName As String)
- Bookmark: id attribute in
<IMG>
element - FileName: The HTML file whose content is to be inserted
Load
Loads the template for processing from the specified HTML file
Public Sub Load(ByVal FileName As String)
SaveAs
Save the current HTML to an HTML file.
Sub SaveAs(FileName As String)
Properties
HTML
Gets the HTML Document’s Element outerHTML. Its most common use is for setting the email HTMLBody property.
Property Get HTML() As String